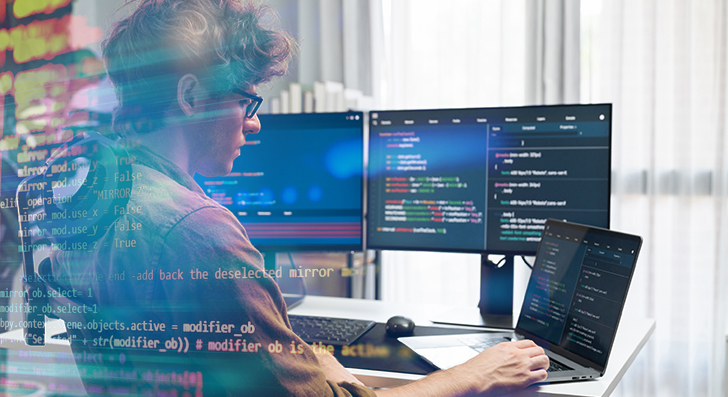
Scalability means your application can take care of development—much more people, far more information, and much more traffic—without the need of breaking. For a developer, creating with scalability in mind will save time and strain later. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not some thing you bolt on afterwards—it should be section of the plan from the beginning. Many apps are unsuccessful every time they expand speedy due to the fact the first layout can’t handle the extra load. To be a developer, you should Believe early regarding how your procedure will behave under pressure.
Start out by creating your architecture being flexible. Stay away from monolithic codebases where by every little thing is tightly connected. As an alternative, use modular design or microservices. These patterns split your application into smaller, impartial sections. Each module or support can scale By itself with out impacting The complete system.
Also, take into consideration your databases from working day a person. Will it require to deal with one million customers or perhaps 100? Select the suitable type—relational or NoSQL—according to how your knowledge will improve. Strategy for sharding, indexing, and backups early, Even though you don’t need to have them still.
A further important stage is to prevent hardcoding assumptions. Don’t compose code that only performs underneath current circumstances. Think of what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use design and style designs that guidance scaling, like concept queues or celebration-pushed programs. These support your app manage a lot more requests without having acquiring overloaded.
Any time you Make with scalability in your mind, you're not just preparing for success—you might be cutting down long run complications. A effectively-prepared technique is easier to maintain, adapt, and mature. It’s improved to arrange early than to rebuild later on.
Use the correct Database
Deciding on the suitable databases is usually a critical Section of creating scalable applications. Not all databases are crafted the exact same, and using the Incorrect you can gradual you down as well as result in failures as your app grows.
Start out by comprehension your information. Can it be hugely structured, like rows inside a desk? If Indeed, a relational database like PostgreSQL or MySQL is a good healthy. They're strong with associations, transactions, and consistency. Additionally they assistance scaling procedures like read through replicas, indexing, and partitioning to handle more website traffic and information.
In the event your info is a lot more flexible—like person activity logs, merchandise catalogs, or documents—take into account a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more conveniently.
Also, contemplate your examine and create designs. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you currently dealing with a major create load? Investigate databases which can deal with substantial create throughput, as well as party-based info storage programs like Apache Kafka (for non permanent data streams).
It’s also wise to Consider in advance. You might not need to have State-of-the-art scaling features now, but choosing a database that supports them implies you gained’t need to have to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your details depending on your access patterns. And usually check database efficiency while you expand.
In a nutshell, the best databases depends on your application’s composition, velocity desires, And exactly how you be expecting it to improve. Acquire time to select correctly—it’ll preserve plenty of issues afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, every compact hold off provides up. Badly created code or unoptimized queries can slow down efficiency and overload your method. That’s why it’s crucial to build economical logic from the beginning.
Commence by creating clean, very simple code. Prevent repeating logic and remove something unnecessary. Don’t choose the most complex Option if an easy 1 is effective. Maintain your functions shorter, centered, and easy to check. Use profiling equipment to locate bottlenecks—locations where by your code can take also long to operate or utilizes far too much memory.
Following, take a look at your databases queries. These frequently gradual points down over the code alone. Ensure each query only asks for the info you actually will need. Stay away from Find *, which fetches every little thing, and in its place pick unique fields. Use indexes to hurry up lookups. And steer clear of undertaking too many joins, Specifically throughout big tables.
In case you notice precisely the same details becoming asked for many times, use caching. Retail outlet the outcomes briefly working with tools like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and tends to make your app far more economical.
Make sure to test with huge datasets. Code and queries that operate great with a hundred records may crash after they have to manage one million.
In short, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These methods enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to handle much more consumers and even more targeted traffic. If almost everything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources enable keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across multiple servers. Instead of a person server accomplishing the many get the job done, the load balancer routes end users to distinct servers according to availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this easy to arrange.
Caching is about storing details briefly so it can be reused immediately. When end users ask for the identical information all over again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical different types of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching minimizes databases load, improves velocity, and would make your app additional efficient.
Use caching for things which don’t change typically. And always be sure your cache is current when info does transform.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your application deal with far more buyers, remain rapidly, and Get better from issues. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you require applications that let your application mature easily. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain hardware or guess long term capability. When site visitors will increase, it is possible to increase extra means with just some clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also offer solutions like managed databases, storage, load balancing, and security equipment. It is possible to target creating your app rather than managing infrastructure.
Containers are another vital Software. A container deals your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app in between environments, from your notebook for the cloud, without having surprises. Docker is the most popular Resource for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes help you regulate them. Gustavo Woltmann blog Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it quickly.
Containers also enable it to be very easy to separate aspects of your app into services. You may update or scale components independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources usually means you'll be able to scale speedy, deploy simply, and recover speedily when problems materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Observe Every thing
In case you don’t keep track of your application, you gained’t know when issues go Erroneous. Checking assists you see how your app is undertaking, location problems early, and make greater conclusions as your application grows. It’s a key Portion of constructing scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—check your app way too. Control just how long it will require for people to load internet pages, how frequently faults materialize, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for important problems. For instance, Should your response time goes above a Restrict or simply a company goes down, you'll want to get notified promptly. This can help you correct troubles quickly, usually prior to users even see.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and facts enhance. With out checking, you’ll overlook indications of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about understanding your process and ensuring it really works nicely, even stressed.
Final Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By coming up with cautiously, optimizing correctly, and utilizing the proper applications, you are able to Make apps that expand effortlessly with out breaking under pressure. Get started little, Assume large, and Make smart.